Ex: Binary Trees
Let's start by creating a basic data type to represent a binary tree.
Recall that a binary tree has two main components: nodes and leaves, where each node leads to either 0, 1, or 2 other nodes, and nodes that lead to no other nodes (i.e. has no children) are called leaves.
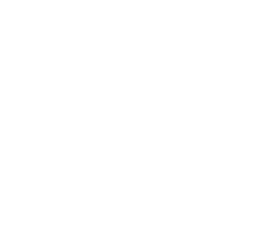
data Empty()
data Leaf(n)
data Node(l, r)
-
Empty()
represents an empty binary tree -
Leaf(n)
represents a leaf with the valuen
-
Node(l,r)
represents a node with childrenl
andr
def size(Empty()) = 0